【jQuery】指定した要素の兄弟要素を取得する方法の一覧!
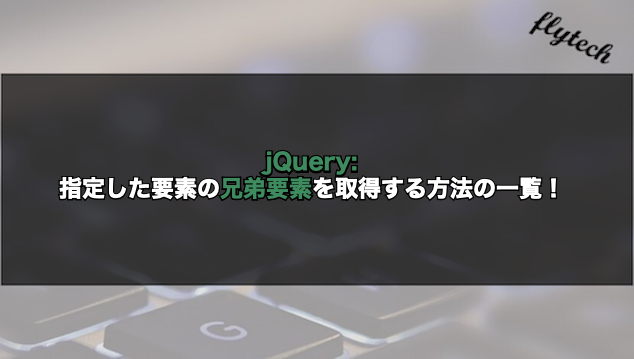
jQueryで指定した要素の兄弟要素を取得するにはnext()系のメソッドやprev()系のメソッド、slice()メソッド、siblings()メソッドなど様々な方法があります。
また、兄弟要素とは、同じ階層にある要素のことをいい、同じ階層にある要素であるならば、指定した要素よりも前の要素でも後ろの要素でも兄弟要素となります。
その為、指定したセレクタから見て、どこの兄弟要素を取得したいのかを確認してから使い分けるようにしましょう。
今回は、指定した要素の兄弟要素を取得する方法の一覧について以下の内容で解説していきます。
⚫︎ 「~」で兄弟要素を取得する場合
⚫︎ next()で兄弟要素を取得する場合
⚫︎ nextAll()で兄弟要素を取得する場合
⚫︎ nextUtill()で兄弟要素を取得する場合
⚫︎ prev()で兄弟要素を取得する場合
⚫︎ prevAll()で兄弟要素を取得する場合
⚫︎ prevUtill()で兄弟要素を取得する場合
⚫︎ siblings()で兄弟要素を取得する場合
⚫︎ slice()で兄弟要素を取得する場合
目次
兄弟要素とは
兄弟要素とは、指定した要素と同じ階層にある要素をいいます。
例えば、グローバルメニューなどのliタグでメニューの項目を表示していますが、liタグから見て、liタグ内の要素全てが兄弟要素になります。
セレクタ関係で指定した要素の兄弟要素を取得する方法
セレクタ関係で指定した要素の兄弟要素を取得する方法を紹介します。
「~」で兄弟要素を取得する場合
「~」を指定して要素の兄弟要素の取得を行ってみます。
sample.html
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 |
<!DOCTYPE html> <html lang="ja"> <head> <meta charset="UTF-8"> <meta name="viewport" content="width=device-width, initial-scale=1.0"> <title>Document</title> <link rel="stylesheet" href="style.css"> <script src="https://ajax.googleapis.com/ajax/libs/jquery/3.5.1/jquery.min.js"></script> </head> <body> <h2>「~」で兄弟要素を取得</h2> <div class= "parent"> <p class= "obj">要素A</p> <p>ここは要素Aの兄弟要素</p> <p>ここは要素Aの兄弟要素</p> <p>ここは要素Aの兄弟要素</p> <p>ここは要素Aの兄弟要素</p> </div> <input type="button" class= "btn1" value="兄弟要素を取得" style= "margin: 5px;"> <script src="index.js"></script> </body> </html> |
index.js
1 2 3 4 5 |
$(function(){ $(".btn1").on("click", function(){ $(".obj~p").css("background", "pink") }); }); |
実行結果
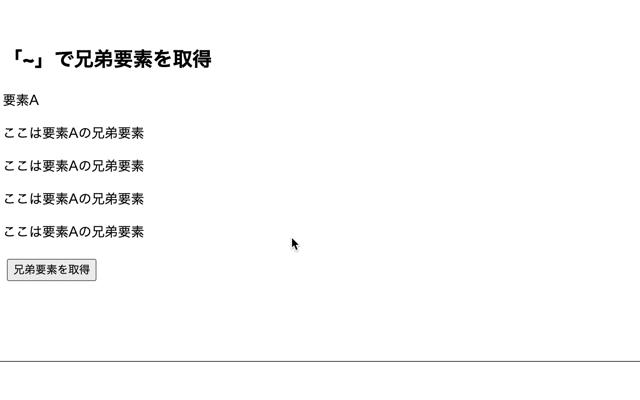
セレクタに「~」を指定することで兄弟要素を取得しています。
メソッドで指定した要素の兄弟要素を取得する方法
next()で兄弟要素を取得する場合
jQueryのnext()メソッドで指定した要素の兄弟要素を取得を行ってみます。
sample.html
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 |
<!DOCTYPE html> <html lang="ja"> <head> <meta charset="UTF-8"> <meta name="viewport" content="width=device-width, initial-scale=1.0"> <title>Document</title> <link rel="stylesheet" href="style.css"> <script src="https://ajax.googleapis.com/ajax/libs/jquery/3.5.1/jquery.min.js"></script> </head> <body> <h2>next()で兄弟要素を取得</h2> <div class= "parent"> <p class= "obj">要素A</p> <p>ここは要素Aの兄弟要素</p> <p>ここは要素Aの兄弟要素</p> <p>ここは要素Aの兄弟要素</p> <p>ここは要素Aの兄弟要素</p> </div> <input type="button" class= "btn1" value="兄弟要素を取得" style= "margin: 5px;"> <script src="index.js"></script> </body> </html> |
index.js
1 2 3 4 5 |
$$(function(){ $(".btn1").on("click", function(){ $(".obj").next().css("background", "orange") }); }); |
実行結果
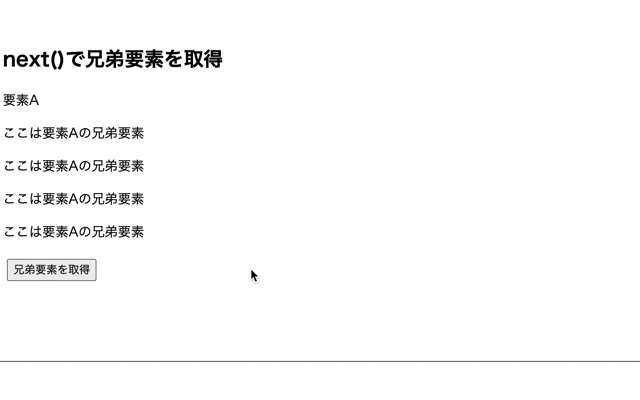
next()メソッドで指定した要素の兄弟要素を取得しています。
jQueryのnext()メソッドついて詳しく知りたい場合はこちらをご参考ください。
nextAll()で兄弟要素を取得する場合
jQueryのnextAll()メソッドで指定した要素の兄弟要素の取得を行ってみます。
sample.html
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 |
<!DOCTYPE html> <html lang="ja"> <head> <meta charset="UTF-8"> <meta name="viewport" content="width=device-width, initial-scale=1.0"> <title>Document</title> <link rel="stylesheet" href="style.css"> <script src="https://ajax.googleapis.com/ajax/libs/jquery/3.5.1/jquery.min.js"></script> </head> <body> <h2>nextAll()で兄弟要素を取得</h2> <div class= "parent"> <p class= "obj">要素A</p> <p>ここは要素Aの兄弟要素</p> <p>ここは要素Aの兄弟要素</p> <p>ここは要素Aの兄弟要素</p> <p>ここは要素Aの兄弟要素</p> </div> <input type="button" class= "btn1" value="兄弟要素を取得" style= "margin: 5px;"> <script src="index.js"></script> </body> </html> |
index.js
1 2 3 4 5 |
$(function(){ $(".btn1").on("click", function(){ $(".obj").nextAll().css("background", "yellow") }); }); |
実行結果
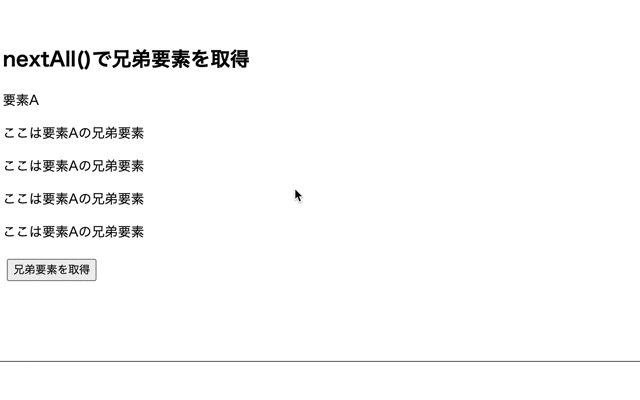
nextAll()メソッドで指定した要素の兄弟要素を取得しています。
jQueryのnextAll()メソッドについて詳しく知りたい場合はこちらをご参考ください。
nextUntil()で兄弟要素を取得する場合
jQueryのnextUntil()メソッドで指定した要素の兄弟要素の取得を行ってみます。
sample.html
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 |
<!DOCTYPE html> <html lang="ja"> <head> <meta charset="UTF-8"> <meta name="viewport" content="width=device-width, initial-scale=1.0"> <title>Document</title> <link rel="stylesheet" href="style.css"> <script src="https://ajax.googleapis.com/ajax/libs/jquery/3.5.1/jquery.min.js"></script> </head> <body> <h2>nextUntil()で兄弟要素を取得</h2> <div class= "parent"> <p class= "obj">要素A</p> <p>ここは要素Aの兄弟要素</p> <p>ここは要素Aの兄弟要素</p> <p>ここは要素Aの兄弟要素</p> <p>ここは要素Aの兄弟要素</p> </div> <input type="button" class= "btn1" value="兄弟要素を取得" style= "margin: 5px;"> <script src="index.js"></script> </body> </html> |
index.js
1 2 3 4 5 |
$(function(){ $(".btn1").on("click", function(){ $(".obj").nextUntil().css("background", "gold") }); }); |
実行結果
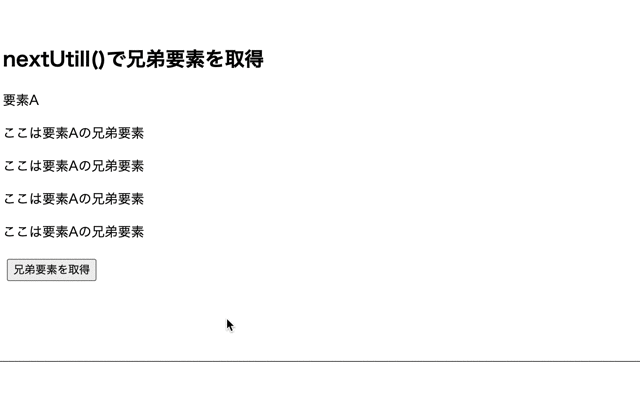
nextUntil()メソッドで指定した要素の兄弟要素を取得しています。
prev()で兄弟要素を取得する場合
jQueryのprev()メソッドで指定した要素の兄弟要素の取得を行ってみます。
sample.html
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 |
<!DOCTYPE html> <html lang="ja"> <head> <meta charset="UTF-8"> <meta name="viewport" content="width=device-width, initial-scale=1.0"> <title>Document</title> <link rel="stylesheet" href="style.css"> <script src="https://ajax.googleapis.com/ajax/libs/jquery/3.5.1/jquery.min.js"></script> </head> <body> <h2>prev()で兄弟要素を取得</h2> <div class= "parent"> <p>ここは要素Aの兄弟要素</p> <p>ここは要素Aの兄弟要素</p> <p>ここは要素Aの兄弟要素</p> <p>ここは要素Aの兄弟要素</p> <p class= "obj">要素A</p> </div> <input type="button" class= "btn1" value="兄弟要素を取得" style= "margin: 5px;"> <script src="index.js"></script> </body> </html> |
index.js
1 2 3 4 5 |
$(function(){ $(".btn1").on("click", function(){ $(".obj").prev().css("background", "blue") }); }); |
実行結果
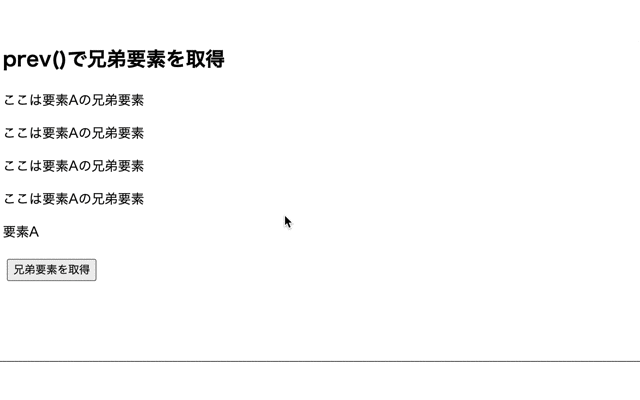
prev()メソッドで指定した要素の兄弟要素を取得しています。
jQueryのprev()メソッドについて詳しく知りたい場合はこちらをご参考ください。
prevAll()で兄弟要素を取得する場合
jQueryのprevAll()メソッドで指定した要素の兄弟要素を取得を行ってみます。
sample.html
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 |
<!DOCTYPE html> <html lang="ja"> <head> <meta charset="UTF-8"> <meta name="viewport" content="width=device-width, initial-scale=1.0"> <title>Document</title> <link rel="stylesheet" href="style.css"> <script src="https://ajax.googleapis.com/ajax/libs/jquery/3.5.1/jquery.min.js"></script> </head> <body> <h2>prevAll()で兄弟要素を取得</h2> <div class= "parent"> <p>ここは要素Aの兄弟要素</p> <p>ここは要素Aの兄弟要素</p> <p>ここは要素Aの兄弟要素</p> <p>ここは要素Aの兄弟要素</p> <p class= "obj">要素A</p> </div> <input type="button" class= "btn1" value="兄弟要素を取得" style= "margin: 5px;"> <script src="index.js"></script> </body> </html> |
index.js
1 2 3 4 5 |
$(function(){ $(".btn1").on("click", function(){ $(".obj").prevAll().css("background", "skyblue") }); }); |
実行結果
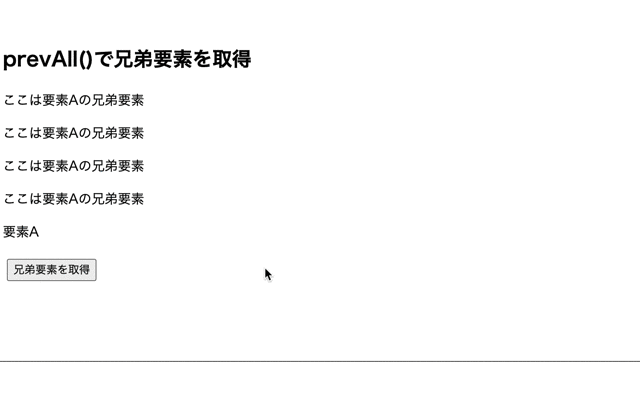
prevAll()メソッドで指定した要素の兄弟要素を取得しています。
prevUntil()で兄弟要素を取得する場合
jQueryのprevUntil()メソッドで指定した要素の兄弟要素を取得を行ってみます。
sample.html
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 |
<!DOCTYPE html> <html lang="ja"> <head> <meta charset="UTF-8"> <meta name="viewport" content="width=device-width, initial-scale=1.0"> <title>Document</title> <link rel="stylesheet" href="style.css"> <script src="https://ajax.googleapis.com/ajax/libs/jquery/3.5.1/jquery.min.js"></script> </head> <body> <h2>prevUntil()で兄弟要素を取得</h2> <div class= "parent"> <p>ここは要素Aの兄弟要素</p> <p>ここは要素Aの兄弟要素</p> <p>ここは要素Aの兄弟要素</p> <p>ここは要素Aの兄弟要素</p> <p class= "obj">要素A</p> </div> <input type="button" class= "btn1" value="兄弟要素を取得" style= "margin: 5px;"> <script src="index.js"></script> </body> </html> |
index.js
1 2 3 4 5 |
$(function(){ $(".btn1").on("click", function(){ $(".obj").prevUntil().css("background", "purple") }); }); |
実行結果
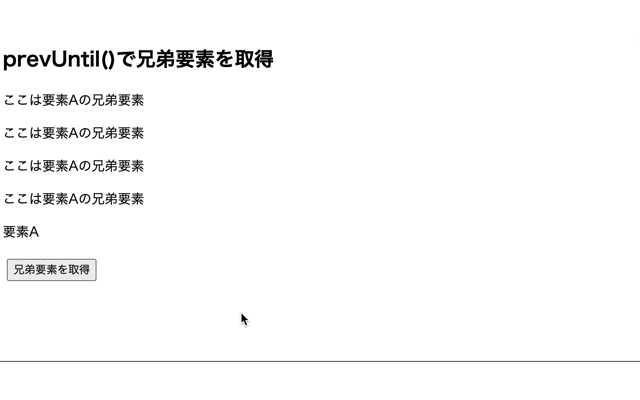
prevUntil()メソッドで指定した要素の兄弟要素を取得しています。
slice()で兄弟要素を取得する場合
jQueryのslice()メソッドで指定した要素の兄弟要素の取得を行ってみます。
sample.html
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 |
<!DOCTYPE html> <html lang="ja"> <head> <meta charset="UTF-8"> <meta name="viewport" content="width=device-width, initial-scale=1.0"> <title>Document</title> <link rel="stylesheet" href="style.css"> <script src="https://ajax.googleapis.com/ajax/libs/jquery/3.5.1/jquery.min.js"></script> </head> <body> <h2>slice()で兄弟要素を取得</h2> <div class= "parent"> <p>要素A</p> <p>ここは要素Aの兄弟要素</p> <p>ここは要素Aの兄弟要素</p> <p>ここは要素Aの兄弟要素</p> <p>ここは要素Aの兄弟要素</p> </div> <input type="button" class= "btn1" value="兄弟要素を取得" style= "margin: 5px;"> <script src="index.js"></script> </body> </html> |
index.js
1 2 3 4 5 |
$(function(){ $(".btn1").on("click", function(){ $("p").slice(1,3).css("background", "brown") }); }); |
実行結果
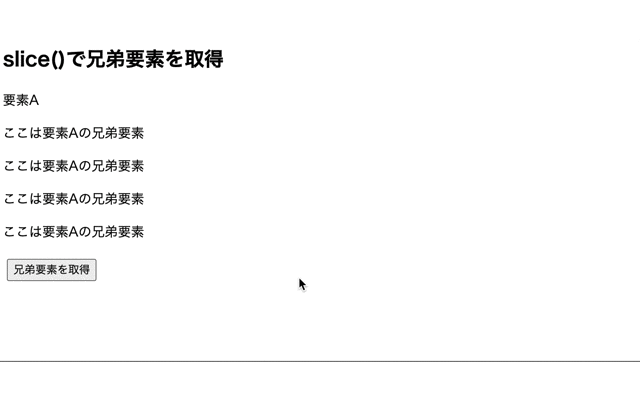
slice()メソッドで指定した要素の兄弟要素を取得しています。
jQueryのslice()メソッドについて詳しく知りたい場合はこちらをご参考ください。
siblings()で兄弟要素を取得する場合
jQueryのsiblings()メソッドで指定した要素の兄弟要素の取得を行ってみます。
sample.html
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 |
<!DOCTYPE html> <html lang="ja"> <head> <meta charset="UTF-8"> <meta name="viewport" content="width=device-width, initial-scale=1.0"> <title>Document</title> <link rel="stylesheet" href="style.css"> <script src="https://ajax.googleapis.com/ajax/libs/jquery/3.5.1/jquery.min.js"></script> </head> <body> <h2>siblings()で兄弟要素を取得</h2> <div class= "parent"> <p>ここは要素Aの兄弟要素</p> <p>ここは要素Aの兄弟要素</p> <p class="obj">要素A</p> <p>ここは要素Aの兄弟要素</p> <p>ここは要素Aの兄弟要素</p> </div> <input type="button" class= "btn1" value="兄弟要素を取得" style= "margin: 5px;"> <script src="index.js"></script> </body> </html> |
index.js
1 2 3 4 5 |
$(function(){ $(".btn1").on("click", function(){ $(".obj").siblings().css("background", "brown") }); }); |
実行結果
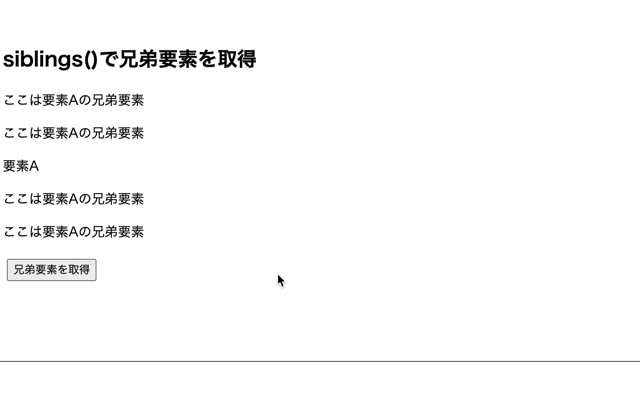
siblings()メソッドで指定した要素の兄弟要素を取得しています。
jQueryのsiblings()メソッドについて詳しく知りたい場合はこちらをご参考ください。
今回のポイント
要素の兄弟要素を取得するには複数のメソッドがある
⚫︎ 兄弟要素とは指定した要素と同じ階層にある要素である
⚫︎ 要素の兄弟要素を取得するにはセレクタに「~」を使用したり、next()、nextAll()、nextUntil()、prev()、prevAll()、slice()、siblings()を使用したりするなど様々な方法がある
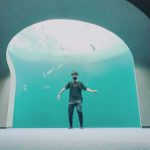
ST
株式会社flyhawkのSTです。フライテックメディア事業部でのメディア運営・ライター業務なども担当。愛機はMac Book AirとThinkPad。好きな言語:swift、JS系(Node.js等)。好きなサーバー:AWS。受託開発やプログラミングスクールの運営をしております。ご気軽にお問い合わせください。
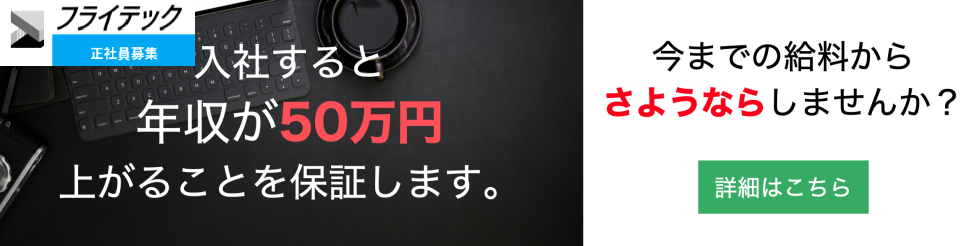