【jQuery】bind()を使って要素にイベントを紐づける!
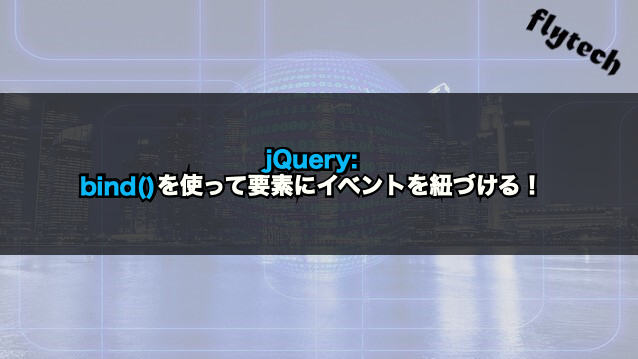
要素にイベントを紐づけるにはjQueryのbind()メソッドを使用します。
jQueryのbind()メソッドとは要素に対してイベントを設定するメソッドであり、ボタンや画像などをクリックした際にイベントを発生させることができます。
イベントを発生させるにはclick()がありますが、bind()メソッドははclick()メソッドと比べて複数のイベントに1つのイベントハンドラを割り当てたり、複数のイベントそれぞれに個々のイベントハンドラを割り当てることができますので複数のイベントを指定する場合はbind()を使用します。
今回は、jQueryのbind()メソッドを使って要素にイベントを紐づけ方法について以下の内容で解説していきます。
⚫︎ 要素に単数のイベントを紐づける場合
⚫︎ 要素に複数のイベントを紐づける場合
⚫︎ 要素にイベントデータを受け渡してイベントを紐づける場合
⚫︎ 複数のイベントそれぞれに個々のイベントハンドラを割り当てる場合
目次
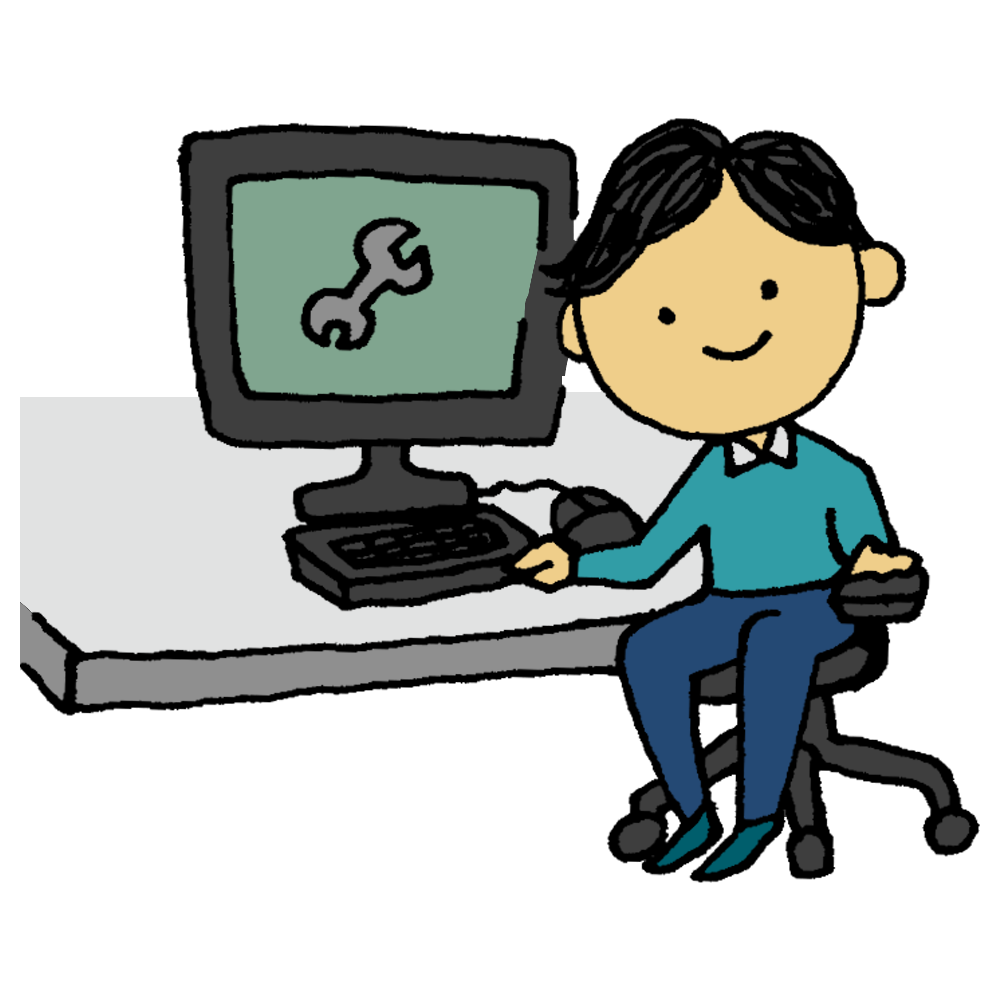
今回はjQueryのbind()メソッドで要素にイベントを紐づける方法について説明していきます。
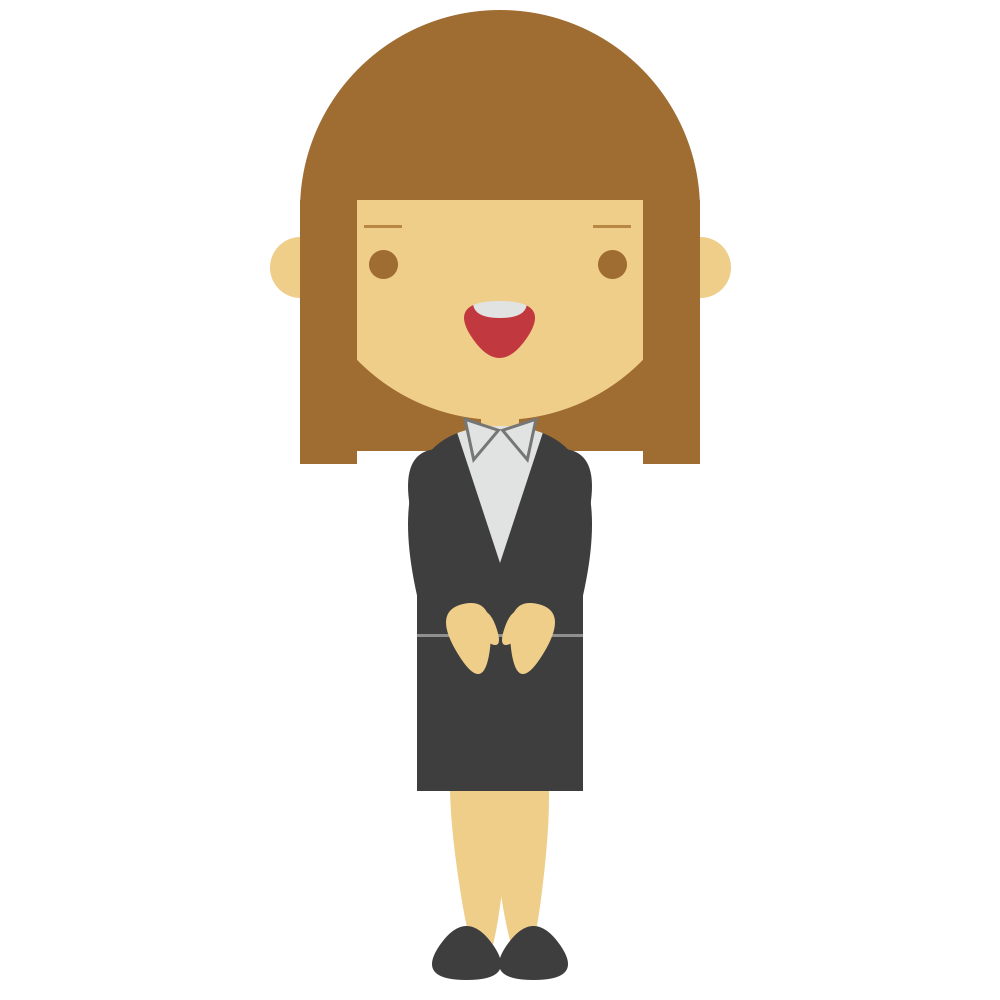
お願いします!
bind()とは
bind()とは、要素に対してイベントを設定するメソッドとなります。
bind()の書き方
bind()メソッドの書き方は下記となります。
1 |
$("セレクタ").bind("イベント名", [オブジェクトデータ], "イベントハンドラ"); |
パラメータ
⚫︎ イベント名:
イベント名を指定する(clickやmouseovereなど)
⚫︎ オブジェクトデータ(任意)
イベントオブジェクトに渡すデータを指定する(オブジェクトリテラル式で指定)
⚫︎ イベントハンドラ:
イベントのアクションを関数で指定する
bind()メソッドのパラメータにイベント名とイベントハンドラを指定することで要素にイベントを紐づけることができます。
また、パラメータにオブジェクトデータも指定すると、イベントオブジェクトにデータ渡した状態で要素にイベントを紐づけることもできます。
要素にイベントを紐づけるサンプルコード
bind()で要素にイベントを紐づけるサンプルコードを紹介します。
要素に単数のイベントを紐づける場合
要素に単数のイベントを紐づける場合はbind()のパラメータにイベント名とイベントハンドラを指定します。
sample.html
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 |
<!DOCTYPE html> <html lang="ja"> <head> <meta charset="UTF-8"> <meta name="viewport" content="width=device-width, initial-scale=1.0"> <title>Document</title> <link rel="stylesheet" href="style.css"> <script src="https://ajax.googleapis.com/ajax/libs/jquery/3.4.1/jquery.min.js"></script> </head> <body> <h2>bind()でmouseoverイベントを紐づける</h2> <div class= "sampleA" id= "sampleD"> </div> <div class= "sampleB" id= "sampleE"> </div> <div class= "sampleC" id= "sampleF"> </div> <p class= "out"></p> <script src="index.js"></script> </body> </html> |
style.css
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 |
.sampleA { width: 100px; height: 100px; background-color: red; } .sampleB { width: 100px; height: 100px; background-color: blue; } .sampleC { width: 100px; height: 100px; background-color: green; } |
index.js
1 2 3 4 5 6 |
$(function(){ $("div").bind("mouseover",function() { let idText = this.id; $(".out").text("id属性名:" + idText); }); }); |
出力結果
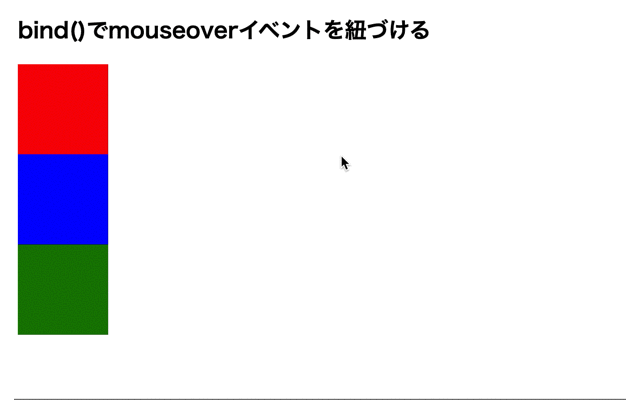
bind()のパラメータにイベント名とイベントハンドラを指定することで要素に単数のイベントを紐づけています。
そのため、マウスを要素の上に持っていくと、各要素のid名が表示されます。
要素に複数のイベントを紐づける場合
bind()で要素に複数のイベントを紐づける場合はbind()メソッドの引数であるイベント名を半角空白空けて指定します。
sample.html
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 |
<!DOCTYPE html> <html lang="ja"> <head> <meta charset="UTF-8"> <meta name="viewport" content="width=device-width, initial-scale=1.0"> <title>Document</title> <link rel="stylesheet" href="style.css"> <script src="https://ajax.googleapis.com/ajax/libs/jquery/3.4.1/jquery.min.js"></script> </head> <body> <h2>bind()でmouseoverイベントとclickイベントを紐づける</h2> <div class= "sampleA" id= "sampleD"> </div> <div class= "sampleB" id= "sampleE"> </div> <div class= "sampleC" id= "sampleF"> </div> <p class= "out"></p> <script src="index.js"></script> </body> </html> |
style.css
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 |
.sampleA { width: 100px; height: 100px; background-color: red; } .sampleB { width: 100px; height: 100px; background-color: blue; } .sampleC { width: 100px; height: 100px; background-color: green; } |
index.js
1 2 3 4 5 6 |
$(function(){ $("div").bind("mouseleave click",function() { let idText = this.id; $(".out").text("id属性名:" + idText); }); }); |
出力結果
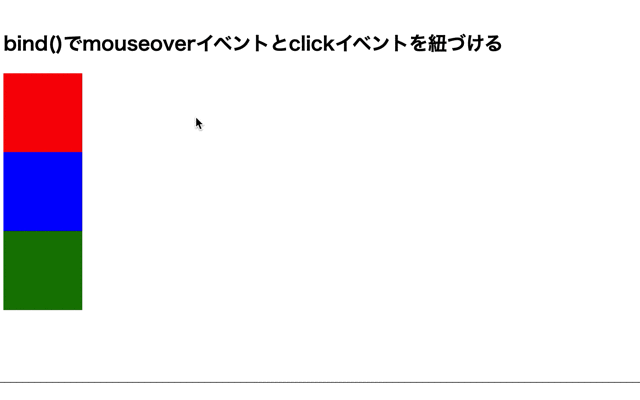
bind()のパラメータにイベント名を半角空白を空けて指定することで要素に複数のイベントを紐づけています。
そのため、マウスを要素の上に持っていく場合と要素からマウスを離した場合には各要素のid名が表示されています。
要素にイベントデータを受け渡してイベントを紐づける場合
bind()で要素にイベントデータを受け渡してイベントを紐づける場合は第2パラメータにオブジェクトデータを指定します。
sample.html
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 |
<!DOCTYPE html> <html lang="ja"> <head> <meta charset="UTF-8"> <meta name="viewport" content="width=device-width, initial-scale=1.0"> <title>Document</title> <link rel="stylesheet" href="style.css"> <script src="https://ajax.googleapis.com/ajax/libs/jquery/3.4.1/jquery.min.js"></script> </head> <body> <h2>bind()で要素にイベントデータを受け渡してイベントを紐づける</h2> <div class= "sampleA" id= "sampleD"> </div> <div class= "sampleB" id= "sampleE"> </div> <div class= "sampleC" id= "sampleF"> </div> <p class= "out"></p> <p class= "out2"></p> <script src="index.js"></script> </body> </html> |
style.css
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 |
.sampleA { width: 100px; height: 100px; background-color: red; } .sampleB { width: 100px; height: 100px; background-color: blue; } .sampleC { width: 100px; height: 100px; background-color: green; } |
index.js
1 2 3 4 5 6 7 8 |
let text = "オブジェクトデータを渡す。" $(function(){ $("div").bind("click", { txt: text }, function(event) { let idText = this.id; $(".out").text("id属性名:" + idText); $(".out2").text("オブジェクトデータ:" + event.data.txt).fadeOut(1000);; }); }); |
出力結果
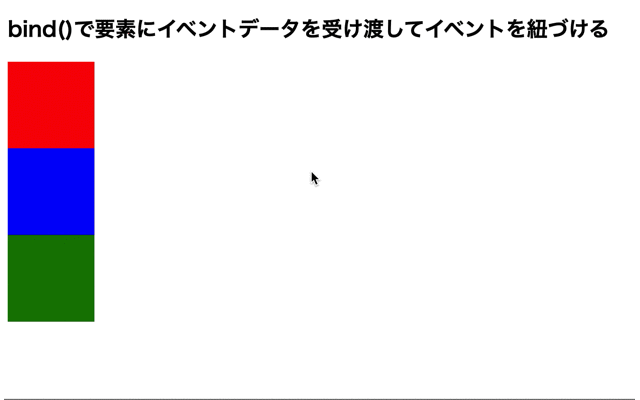
bind()で、第2パラメータにオブジェクトデータを指定することで要素にイベントデータを受け渡してイベントを紐づけることができます。
そのため、要素をクリックすると、第2パラメータで指定したオブジェクトデータをイベントハンドラに渡して表示されています。
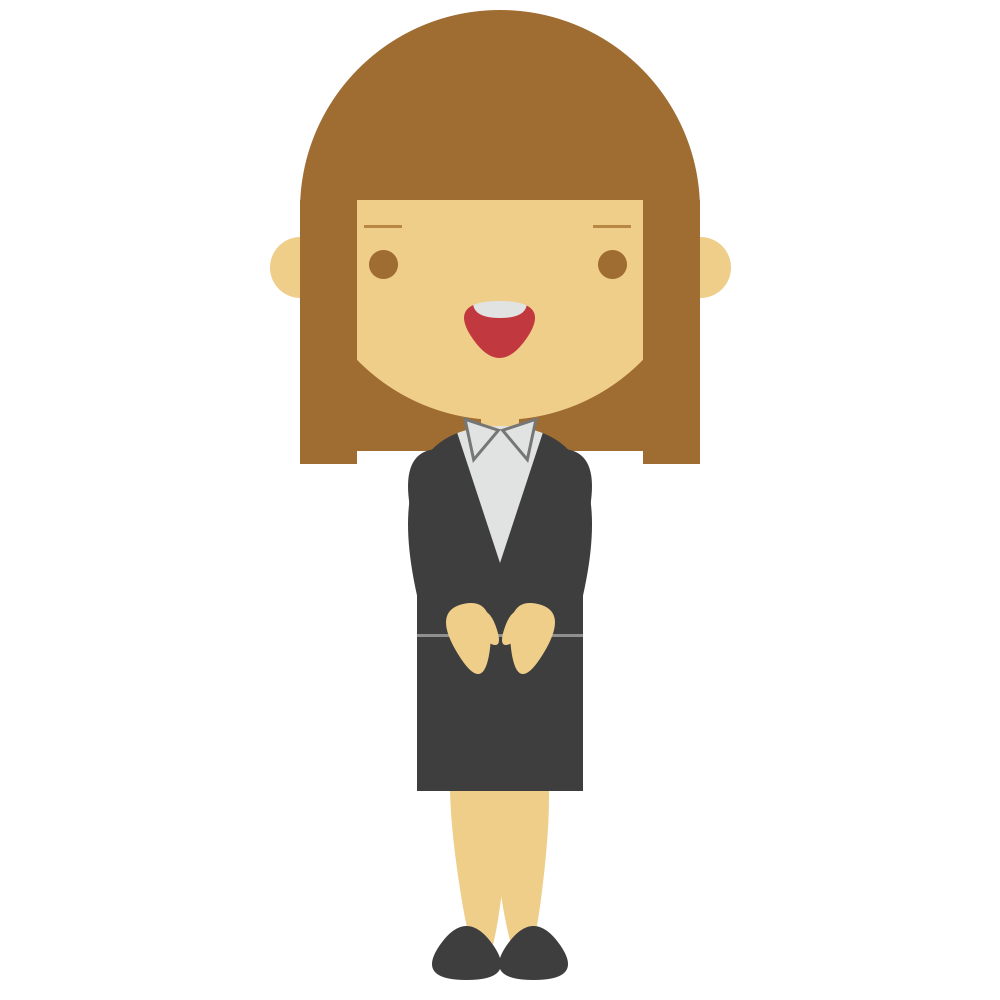
bind()の第2パラメータにオブジェクトデータを指定することで要素にイベントデータを受け渡してイベントを紐づけることができるんですね!
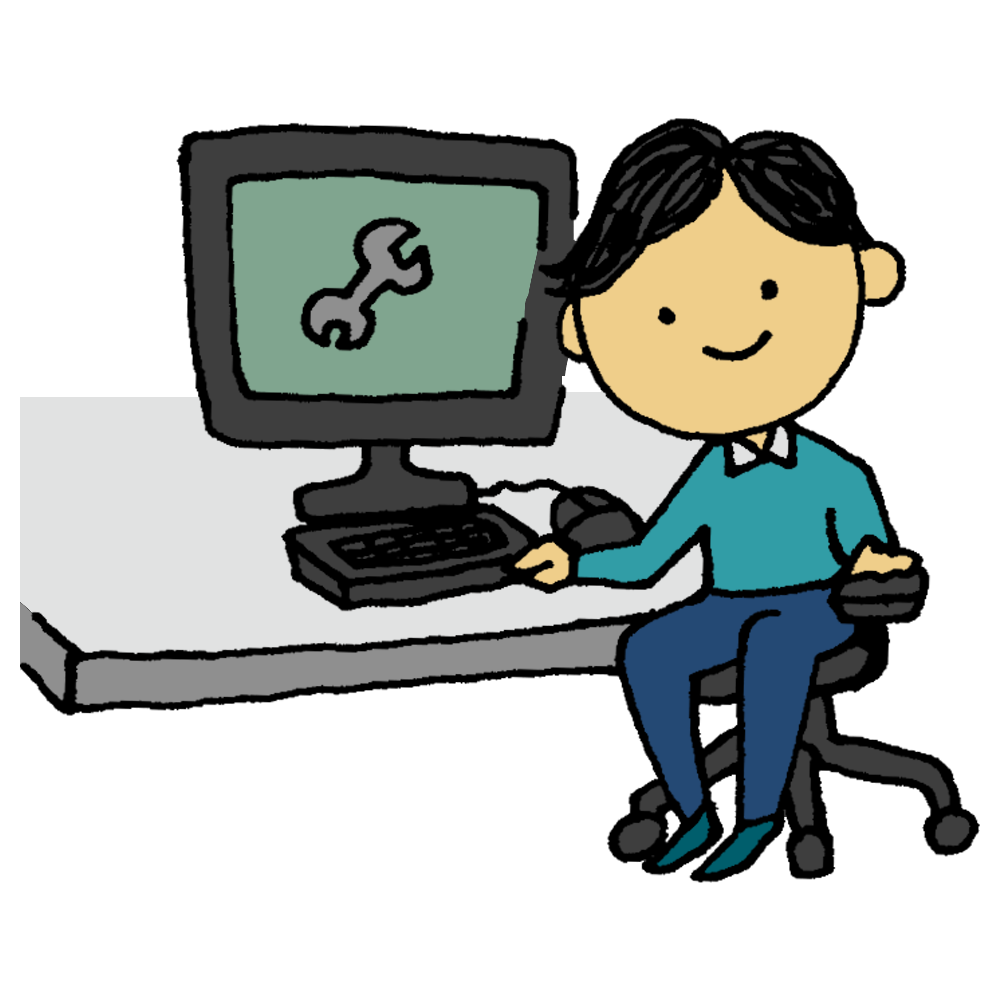
そうですね。
bind()はパラメータが2つの場合と3つの場合がありますが基本的にはパラメータを2で使用する場合が多いかと思います。
複数のイベントそれぞれに個々のイベントハンドラを割り当てる場合
複数のイベントそれぞれに個々のイベントハンドラを割り当てる場合はbind()メソッドのパラメータをマップで指定します。
sample.html
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 |
<!DOCTYPE html> <html lang="ja"> <head> <meta charset="UTF-8"> <meta name="viewport" content="width=device-width, initial-scale=1.0"> <title>Document</title> <link rel="stylesheet" href="style.css"> <script src="https://ajax.googleapis.com/ajax/libs/jquery/3.4.1/jquery.min.js"></script> </head> <body> <h2>bind()で複数のイベントそれぞれに個々のイベントハンドラを割り当てる</h2> <div class= "sampleA" id= "sampleD"> </div> <div class= "sampleB" id= "sampleE"> </div> <div class= "sampleC" id= "sampleF"> </div> <script src="index.js"></script> </body> </html> |
style.css
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 |
.sampleA { width: 100px; height: 100px; background-color: red; } .sampleB { width: 100px; height: 100px; background-color: blue; } .sampleC { width: 100px; height: 100px; background-color: green; } |
index.js
1 2 3 4 5 6 7 8 9 10 11 |
$(function(){ $("div").bind({click: function() { $(this).css("background", "gray"); }, mouseover: function() { $(this).css("background", "black"); }, mouseout: function() { $(this).css("background", "skyblue"); }}); }); |
出力結果
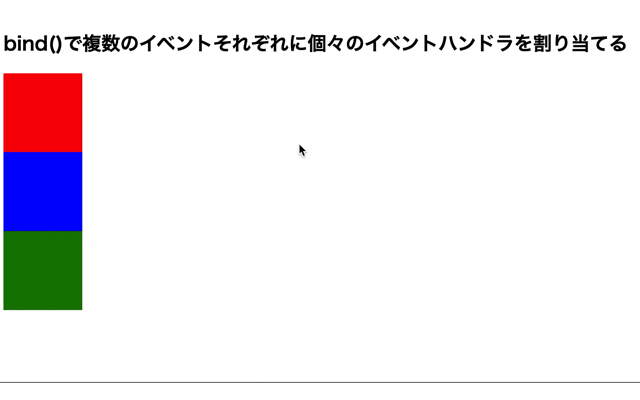
bind()メソッドのパラメータをマップで指定することで複数のイベントそれぞれに個々のイベントハンドラを割り当てることができます。
そのため、要素をクリックすると灰色になり、マウスを持っていくと黒色になり、マウスが離れると水色になります。
今回のポイント
bind()で要素にイベントを紐づける
⚫︎ 要素にイベントを紐づけるにはjQueryのbind()メソッドを使用する
⚫︎ 要素に単数のイベントを紐づける場合はbind()のパラメータにイベント名とイベントハンドラを指定する
⚫︎ bind()で要素に複数のイベントを紐づける場合はbind()メソッドの引数であるイベント名を半角空白空けて指定する
⚫︎ bind()で要素にイベントデータを受け渡してイベントを紐づける場合は第2パラメータにオブジェクトデータを指定する
⚫︎ 複数のイベントそれぞれに個々のイベントハンドラを割り当てる場合はbind()メソッドのパラメータをマップで指定する
関連記事
jQueryのon()メソッドで要素にイベントを設定する方法についてはこちらをご参考ください。
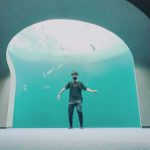
ST
株式会社flyhawkのSTです。フライテックメディア事業部でのメディア運営・ライター業務なども担当。愛機はMac Book AirとThinkPad。好きな言語:swift、JS系(Node.js等)。好きなサーバー:AWS。受託開発やプログラミングスクールの運営をしております。ご気軽にお問い合わせください。
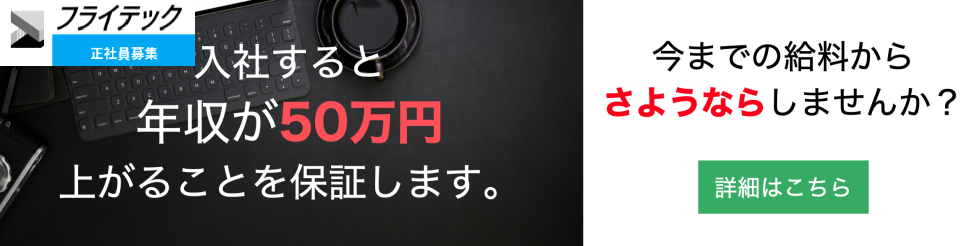